class Media{
private:
int id;
string title;
int year;
public:
Media(){ id = year = 0; title = ""; }
Media(int _id, string _title, int _year): id(_id), title(_title), year(_year) {}
// virtual ~Media() = 0;
void changeID(int newID){ id = newID; }
virtual void print(ostream &out);
};
The thing is: without the destructor, GCC gives me a bunch of warnings class has virtual functions but non-virtual destructor, but still compiles and my program works fine. Now I want to get rid of those annoying warnings so I satisfy the compiler by adding a virtual destructor, the result is: it doesn't compile, with the error:
undefined reference to `Media::~Media()`
Making the destructor pure virtual doesn't solve the problem. So what has gone wrong?
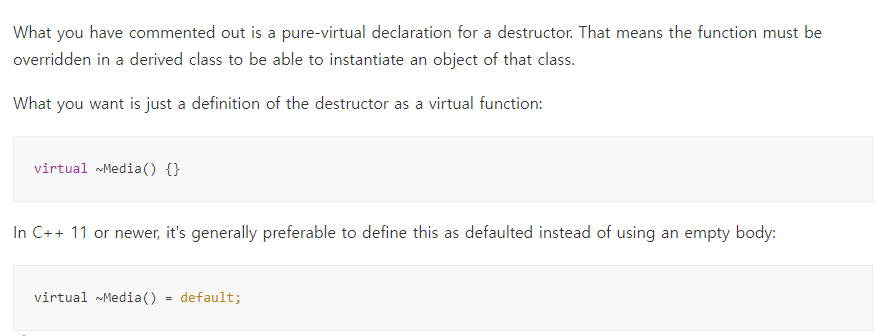
What you have commented out is a pure-virtual declaration for a destructor. That means the function must be overridden in a derived class to be able to instantiate an object of that class.
What you want is just a definition of the destructor as a virtual function:
virtual ~Media() {}
In C++ 11 or newer, it's generally preferable to define this as defaulted instead of using an empty body:
virtual ~Media() = default;
ref : https://stackoverflow.com/questions/10024796/c-virtual-functions-but-no-virtual-destructors
'프로그래밍(Programming) > c++, 11, 14 , 17, 20' 카테고리의 다른 글
using shared_ptr with pure virtual base class (0) | 2022.08.24 |
---|---|
C++ 락(std::lock, std::unique_lock, std::lock_guard, condition_variable...) (0) | 2022.08.11 |
error C4596: illegal qualified name in member (0) | 2022.03.03 |
[Modern C++] std::move , 간단 예시 (0) | 2022.03.03 |
std::forward (0) | 2022.03.03 |