Sol : When you need to hit the ground running with Lua and C++, sol is the go-to framework for high-performance binding with an easy to use API.
GitHub - ThePhD/sol2: Sol3 (sol2 v3.0) - a C++ <-> Lua API wrapper with advanced features and top notch performance - is here, a
Sol3 (sol2 v3.0) - a C++ <-> Lua API wrapper with advanced features and top notch performance - is here, and it's great! Documentation: - GitHub - ThePhD/sol2: Sol3 (sol2 v3.0) - a C++ &l...
github.com
the basics:
Note
The code below and more examples can be found in the examples directory.
#define SOL_ALL_SAFETIES_ON 1
#include <sol/sol.hpp>
#include <assert.hpp>
int main() {
sol::state lua;
int x = 0;
lua.set_function("beep", [&x]{ ++x; });
lua.script("beep()");
c_assert(x == 1);
sol::function beep = lua["beep"];
beep();
c_assert(x == 2);
return 0;
}
#define SOL_ALL_SAFETIES_ON 1
#include <sol/sol.hpp>
#include <assert.hpp>
struct vars {
int boop = 0;
int bop () const {
return boop + 1;
}
};
int main() {
sol::state lua;
lua.new_usertype<vars>("vars",
"boop", &vars::boop,
"bop", &vars::bop);
lua.script("beep = vars.new()\n"
"beep.boop = 1\n"
"bopvalue = beep:bop()");
vars& beep = lua["beep"];
int bopvalue = lua["bopvalue"];
c_assert(beep.boop == 1);
c_assert(lua.get<vars>("beep").boop == 1);
c_assert(beep.bop() == 2);
c_assert(bopvalue == 2);
return 0;
}
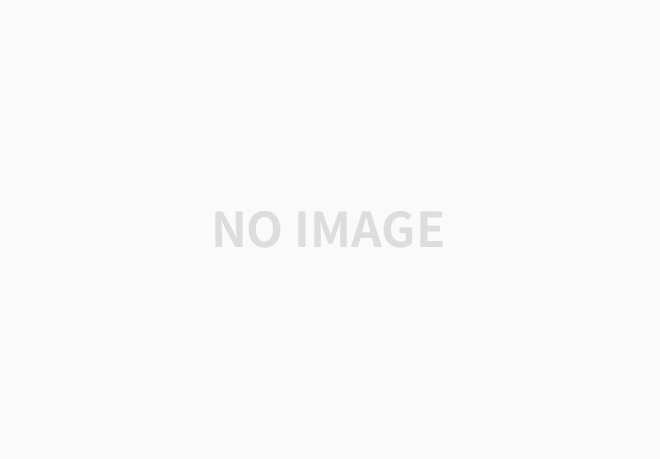
반응형
'프로그래밍(Programming) > Script, lua' 카테고리의 다른 글
Lua : property (0) | 2021.10.13 |
---|---|
Lua 한페이지로 배우기 (0) | 2021.06.24 |
lua tinker에서 구조체를 C 함수에 넘겨 주려면 어떻게 해야 하나요? (0) | 2014.02.24 |